ConnectWallet
ConnectWallet component renders a button which when clicked opens a modal to allow users to connect to wallets specified in the ThirdwebProvider's supportedWallets prop.
If supportedWallets
is not configured in the ThirdwebProvider
, the ConnectWallet Modal shows the below shown default wallets:
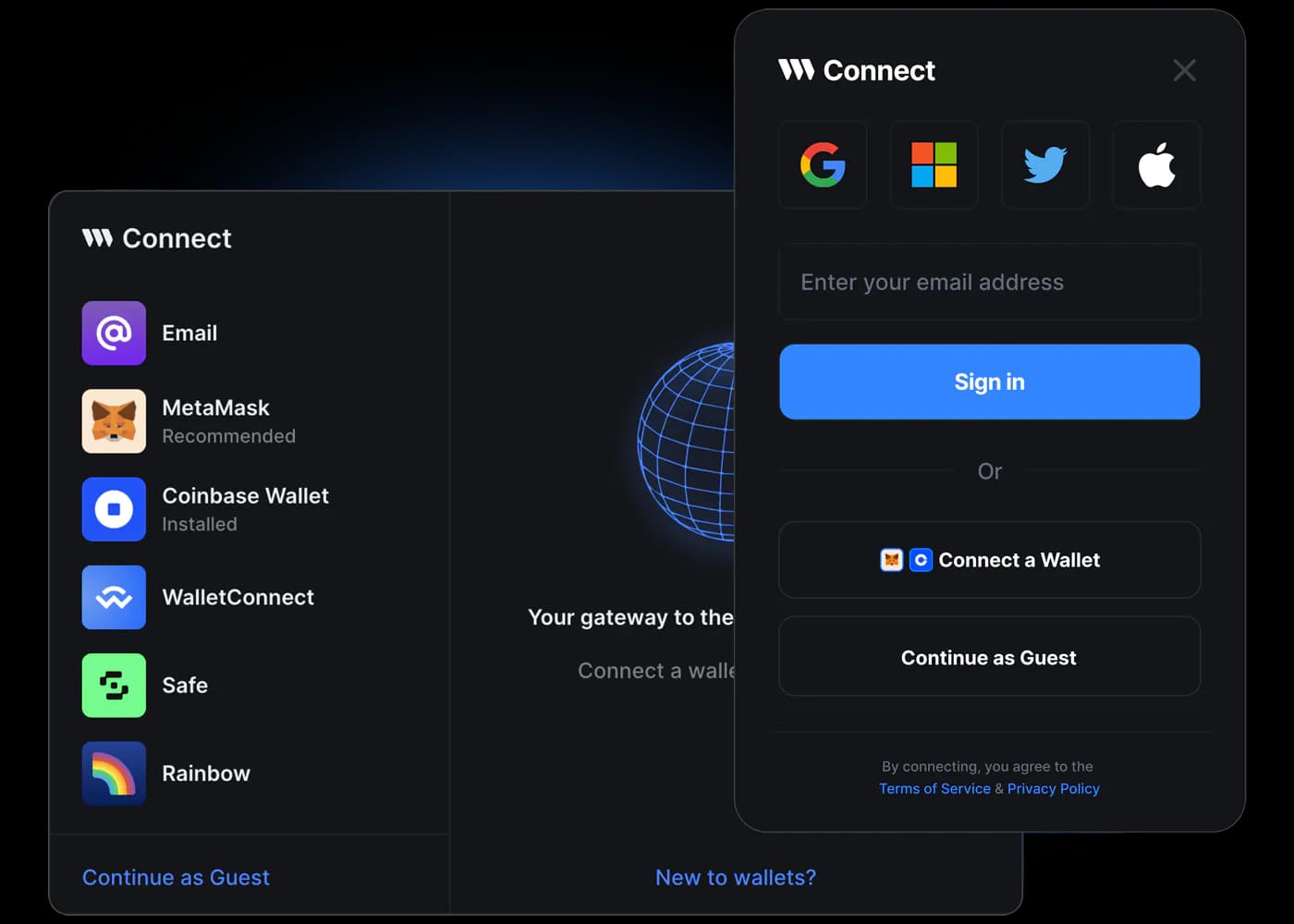
import { ConnectWallet } from "@thirdweb-dev/react-native";function App() {return <ConnectWallet />;}
Playground
Try out ConnectWallet in action on ConnectWallet Playground!
Set the theme to either "light"
or "dark"
or pass a custom theme object to completely customize the look and feel of the ConnectWallet Modal.
The default is "dark"
<ConnectWallet theme="dark" />;
You can fully customize the theme:
import { ConnectWallet, darkTheme } from "@thirdweb-dev/react-native";function App() {return (<ConnectWallettheme={darkTheme({borderRadii: {md: 12,},colors: {accentButtonColor: "black",},buttonTextColor: "white",})}/>);}
Change the text on button when the ConnectWallet button is in the disconnected state.
The default is "Connect Wallet"
<ConnectWallet buttonTitle="Login" />;
Change the title of ConnectWallet Modal
The default is "Connect"
<ConnectWallet modalTitle="Login" />;
Render a custom button to display connected wallet details instead of the default one
<ConnectWalletdetailsButton={() => {return <Button />;}}/>;
Hide the "Request Testnet funds" link in ConnectWallet details modal when user is connected to a testnet.
Default is true
, If you want to show the link, set it to false
.
<ConnectWallet hideTestnetFaucet={false} />;
You can show a "Terms of Service" and/or "Privacy Policy" link in the ConnectWallet Modal by passing the termsOfServiceUrl
and/or privacyPolicyUrl
props
<ConnectWallettermsOfServiceUrl="https://...."privacyPolicyUrl="https://...."/>;
Customize the tokens shown in the "Send Funds" screen for various networks.
By default, The "Send Funds" screen shows a few popular tokens for default chains and the native token. For other chains it only shows the native token.
supportedTokens
prop allows you to customize this list as shown below.
import { Base } from "@thirdweb-dev/chains";const supportedTokens = {// use chain id of the network as key and pass an array of tokens to show// you can directly pass the number or import the chain object from @thirdweb-dev/chains to get the chain id[Base.chainId]: [{address: "0x50c5725949A6F0c72E6C4a641F24049A917DB0Cb", // token contract addressname: "Dai Stablecoin",symbol: "DAI",icon: "https://assets.coingecko.com/coins/images/9956/small/Badge_Dai.png?1687143508",},],};// Show "Dai Stablecoin" when connected to the "Base" mainnetfunction Example() {return <ConnectWallet supportedTokens={supportedTokens} />;}
Display the balance of a token instead of the native token in ConnectWallet details button.
import { Base } from "@thirdweb-dev/chains";// show Wrapped BTC balance when connected to Ethereum mainnet// Show Dai Stablecoin balance when connected to the Base mainnetconst displayBalanceToken = {// 1 is chain id of Ethereum mainnet1: "0x2260FAC5E5542a773Aa44fBCfeDf7C193bc2C599", // contract address of Wrapped BTC token// you can also import the chain object from @thirdweb-dev/chains to get the chain id[Base.chainId]: "0x50c5725949A6F0c72E6C4a641F24049A917DB0Cb", // contract address of Dai Stablecoin token};// pass an object with chain id as key and token address as valuefunction Example() {return <ConnectWallet displayBalanceToken={displayBalanceToken} />;}
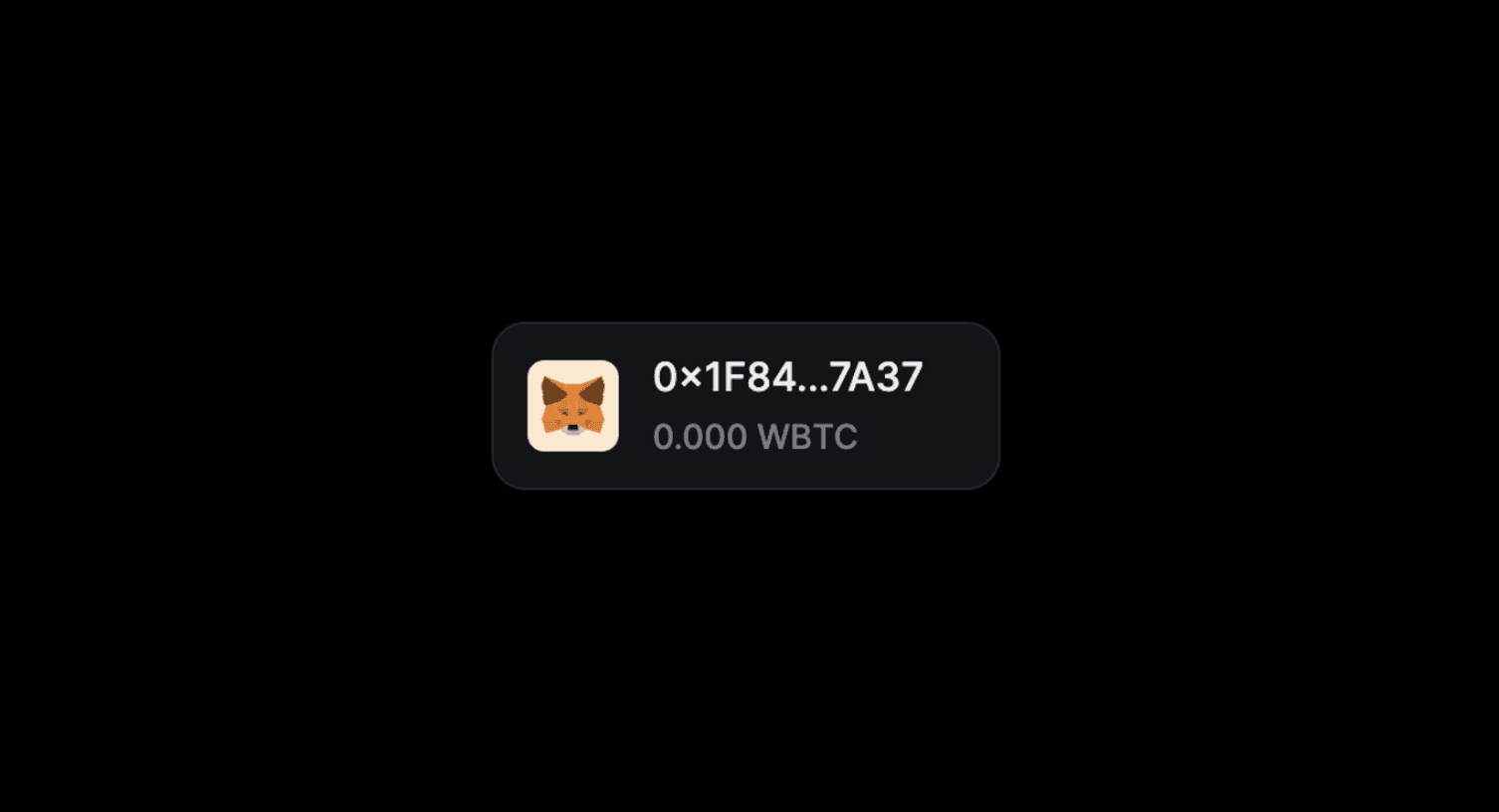
Specify whether to show a "Switch Network" button after the wallet is connected and it is not connected to the activeChain set in ThirdwebProvider to encourage the user to switch to the correct network in their wallet.
activeChain must be explicitly set in ThirdwebProvider for this to work.
default is false
.
<ConnectWallet switchToActiveChain={true} />;
We support localization of our SDK with support for a few languages.
You can pass a locale
prop to our ThirdwebProvider
, see more info in Localizing our UI components.